I often make the argument that frontend developers should focus on re-using components instead of re-using classes. This stems from my belief that components are the superior unit of composition.
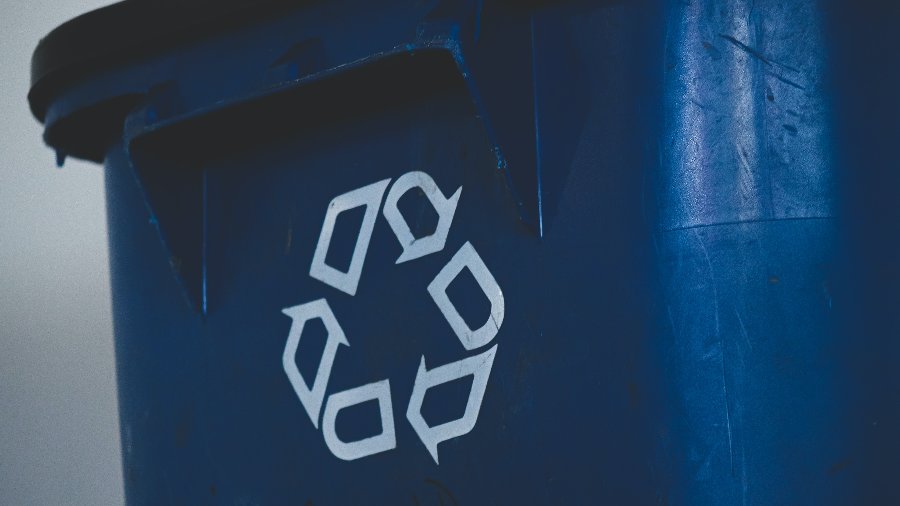
The component model was a game changer in frontend development. It allowed you to encapsulate behavior, structure, and styling all in one re-usable block. This is a very simple but powerful way to re-use code. Many developers would agree with this, yet I don’t believe we are fully using components to their potential when it comes to styling.
I think we are attempting to make classes re-usable when we don’t need to. Instead, we should make components re-usable. Let me explain with an example.
Method 1 (re-using classes)
.big-text {
font-size: 32px;
}
.primary {
color: blue;
}
<div className="big-text primary">Here is some big decorative text</div>
<div className="big-text">Here is some other big text</div>
Method 2 (re-using components)
<Text size="lg" color="primary">Here is some big decorative text</Text>
<Text size="lg">Here is some other big text</Text>
Why is method 2 better?
- There’s no need to go hunting for the
big-text
andprimary
classes to see what styles they apply. It’s very obvious whatsize="lg"
andcolor="primary"
do. Who knows what stylesbig-text
andprimary
apply? They could do any number of things unless we go searching for where they are defined. Also, it’s not always easy to find where these classes are defined. - If
Text
is written in typescript, we will be given auto-complete when adding thesize
andcolor
props. Don’t underestimate how much of a productivity boost auto-complete is. - It’s now up to
Text
to decide how to style the underlying dom node. We’re hiding away this complexity and allowing our styling strategy to change if needed. - Everything is just generally more expressive and easier to read.
Text
is a better name thandiv
.size="lg"
is more expressive syntax thanclassName="big-text"
- We can enable responsive array syntax if we want:
size={["md", "lg"]}
. This will make the size"md"
on mobile and"lg"
on screens larger than mobile. This is a very simple syntax with a powerful effect. Imagine how you would do that with classes while keeping it just as expressive.
In the age of components I don’t see any reason to be using classes except when implementing low-level re-usable components like the Text
example above.
In other words, re-use components instead of classes. Just use classes as a tool to make re-usable components.